Getting Started with Network Data#
import networkx as nx
import matplotlib.pyplot as plt
NetworkX reference page: https://networkx.org/documentation/stable/index.html
Create a graph by yourself#
g = nx.Graph()
# g.add_node(1) # Add a single node
g.add_nodes_from([1,2,3]) # Add a list of nodes
g.add_edge(1,2)
g.add_edge(3,1)
nx.draw_networkx(g)
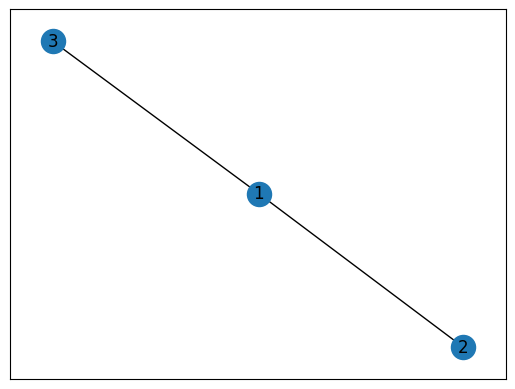
Import a classic network#
g_kk = nx.krackhardt_kite_graph()
nx.draw_networkx(g_kk)
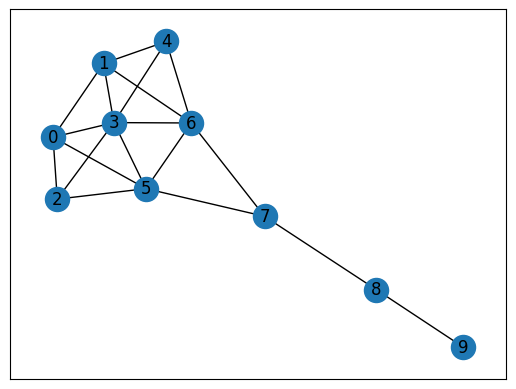
Import a graph file#
In this example, we import a dataset about collaborations in network science. Read more about the network here.
First, download a zip file containing the data from this page. The dataset you need is about Coauthorships in network science. After downloading, open the zip file to unzip it to a directory that you can access.
If you run the notebook locally, change the PATH_TO
below to point the path to the .gml file.
If you use Google Colab, read this page to understand how to upload local files to Colab. The simplest solution is probably to use files.upload()
.
G = nx.read_gml("PATH_TO/netscience.gml") # change PATH_TO
---------------------------------------------------------------------------
FileNotFoundError Traceback (most recent call last)
Cell In[5], line 1
----> 1 G = nx.read_gml("PATH_TO/netscience.gml") # change PATH_TO
File ~/.pyenv/versions/3.11.8/lib/python3.11/site-packages/networkx/utils/decorators.py:789, in argmap.__call__.<locals>.func(_argmap__wrapper, *args, **kwargs)
788 def func(*args, __wrapper=None, **kwargs):
--> 789 return argmap._lazy_compile(__wrapper)(*args, **kwargs)
File <class 'networkx.utils.decorators.argmap'> compilation 34:3, in argmap_read_gml_29(path, label, destringizer, backend, **backend_kwargs)
1 import bz2
2 import collections
----> 3 import gzip
4 import inspect
5 import itertools
File ~/.pyenv/versions/3.11.8/lib/python3.11/site-packages/networkx/utils/decorators.py:199, in open_file.<locals>._open_file(path)
195 else:
196 # could be None, or a file handle, in which case the algorithm will deal with it
197 return path, lambda: None
--> 199 fobj = _dispatch_dict[ext](path, mode=mode)
200 return fobj, lambda: fobj.close()
FileNotFoundError: [Errno 2] No such file or directory: 'PATH_TO/netscience.gml'
list(G.nodes(data=True))
list(G.edges(data=True))
nx.draw_networkx(G, with_labels=False)
Import an edge list file#
import pandas as pd
df = pd.read_csv("https://raw.githubusercontent.com/eehh-stanford/SNA-workshop/master/hp5edgelist.txt", delimiter='\t')
df = df.rename(columns={"“From”": "From", '“To”': "To"})
df
DG = nx.from_pandas_edgelist(df, 'From', 'To', create_using=nx.DiGraph())
nx.draw_networkx(DG)
nx.density(DG)
list(nx.degree(DG))
Other datasets#
If you are interested in playing with more datasets, there are many places to find network data. Below is a list of sites you can start with:
Have fun!